Wevr
How to use Automated Trading
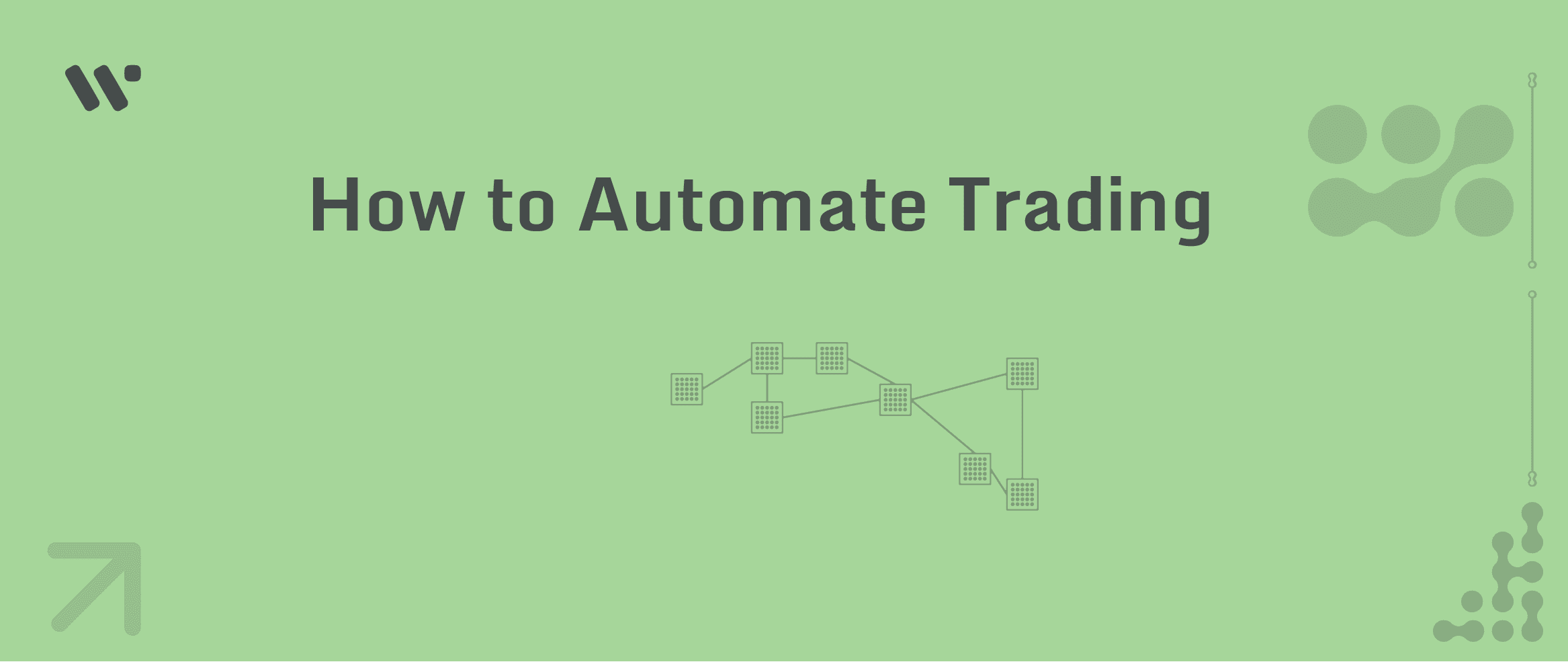
Automating Cryptocurrency Trading with On-chain Metrics
Disclaimer: This article is for educational purposes only and does not constitute financial advice. Cryptocurrency trading involves significant risks, and automated trading systems can amplify these risks. Always conduct thorough research and consider consulting with a financial advisor before engaging in any trading activities.
In the fast-paced world of cryptocurrency trading, automation can be a powerful tool to execute strategies consistently and efficiently. By leveraging on-chain metrics, traders can create data-driven algorithms that respond to real-time blockchain activity. This article will explore how to automate trading using on-chain metrics, with a focus on using Python to implement these strategies.
Understanding On-chain Metrics for Automation
Before diving into automation, it's crucial to understand which on-chain metrics can be effectively used in trading algorithms. Some key metrics include:
- Transaction Volume
- Active Addresses
- Network Hash Rate
- Gas Fees (for networks like Ethereum)
- Token Transfers (especially large "whale" movements)
- Exchange Inflows and Outflows
Setting Up Your Python Environment
To get started with automated trading using on-chain metrics, you'll need to set up a Python environment with the necessary libraries. Here's a basic setup - Keep in mind this is boilerplate:
import pandas as pd
import numpy as np
import requests
from web3 import Web3
import ccxt
These libraries will help you fetch data, perform calculations, interact with blockchain networks, and execute trades.
Fetching On-chain Data
The first step in automating your trading strategy is to fetch relevant on-chain data. Here's an example of how you might fetch transaction volume data for Ethereum using the Etherscan API:
def get_eth_transaction_volume():
url = "https://api.etherscan.io/api"
params = {
"module": "proxy",
"action": "eth_blockNumber",
"apikey": "YOUR_API_KEY"
}
response = requests.get(url, params=params)
latest_block = int(response.json()["result"], 16)
params["action"] = "eth_getBlockByNumber"
params["tag"] = hex(latest_block)
params["boolean"] = "true"
response = requests.get(url, params=params)
block_data = response.json()["result"]
return int(block_data["totalDifficulty"], 16)
# Example usage
eth_volume = get_eth_transaction_volume()
print(f"Current Ethereum transaction volume: {eth_volume}")
Implementing a Simple Trading Strategy
Now, let's implement a basic trading strategy based on transaction volume. This strategy will buy when the volume increases significantly and sell when it decreases:
def simple_volume_strategy(current_volume, average_volume, threshold=1.5):
if current_volume > average_volume * threshold:
return "BUY"
elif current_volume < average_volume / threshold:
return "SELL"
else:
return "HOLD"
# Initialize variables
volume_history = []
trading_signal = "HOLD"
# Main trading loop
while True:
current_volume = get_eth_transaction_volume()
volume_history.append(current_volume)
if len(volume_history) > 24: # Assuming we're collecting hourly data
average_volume = np.mean(volume_history[-24:])
trading_signal = simple_volume_strategy(current_volume, average_volume)
print(f"Current Signal: {trading_signal}")
# Here you would implement actual trading logic based on the signal
# This could involve interacting with an exchange API to place orders
time.sleep(3600) # Wait for an hour before the next check
Integrating with an Exchange
To execute trades based on your signals, you'll need to integrate with a cryptocurrency exchange. Here's a basic example using the CCXT library to place a market buy order:
def place_market_buy_order(symbol, amount):
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
})
try:
order = exchange.create_market_buy_order(symbol, amount)
print(f"Order placed: {order}")
except Exception as e:
print(f"An error occurred: {e}")
# Example usage
if trading_signal == "BUY":
place_market_buy_order('ETH/USDT', 0.1) # Buy 0.1 ETH
Advanced Considerations
While the above examples provide a starting point, there are many factors to consider when building a robust automated trading system:
-
Risk Management: Implement stop-loss and take-profit mechanisms to manage risk.
-
Multiple Indicators: Combine multiple on-chain metrics for more sophisticated decision-making.
-
Backtesting: Test your strategy on historical data before running it live.
-
Error Handling: Implement robust error handling and logging to manage potential issues.
-
Regulatory Compliance: Ensure your trading bot complies with relevant regulations in your jurisdiction.
-
Security: Protect your API keys and implement secure practices to prevent unauthorized access.
Wevr.ai API
In the future quarter Wevr plans to release our API which can be hooked into your automated trading strategies to assist with decision making!
Conclusion
Automating cryptocurrency trading using on-chain metrics can be a powerful way to leverage blockchain data for potential trading advantages. However, it's crucial to approach this with caution, thorough testing, and a deep understanding of both the technology and the risks involved.
At Wevr, we understand the complexities of the crypto market and the importance of reliable, real-time data. Our advanced on-chain analytics and whale tracking capabilities can provide valuable insights to enhance your automated trading strategies.
Remember, the code examples provided here are simplified for educational purposes and should not be used for actual trading without significant enhancements and risk management strategies. Wevr's suite of tools can help you develop more robust and informed trading algorithms, but it's essential to use these resources responsibly.
As you develop your automated trading system, continually educate yourself about blockchain technology, programming best practices, and financial risk management. We encourage our users to leverage the extensive educational materials available at Wevr's Crypto insights blog to stay informed about the latest developments in the crypto space.
The cryptocurrency market is highly volatile and complex, and even the most sophisticated algorithms can't guarantee profits. That's why it's crucial to have access to comprehensive market insights and powerful analytical tools like those offered by Wevr.
Stay ahead of the curve by visiting Wevr.ai and exploring our range of services tailored to enhance your trading strategies. Join our community on Twitter to keep up with the latest insights and updates.
Remember, in the world of automated crypto trading, knowledge and data are power. Stay informed, stay analytical, and let Wevr be your guide through the exciting and often unpredictable world of algorithmic cryptocurrency trading.
Happy coding, and trade responsibly!
Related Posts
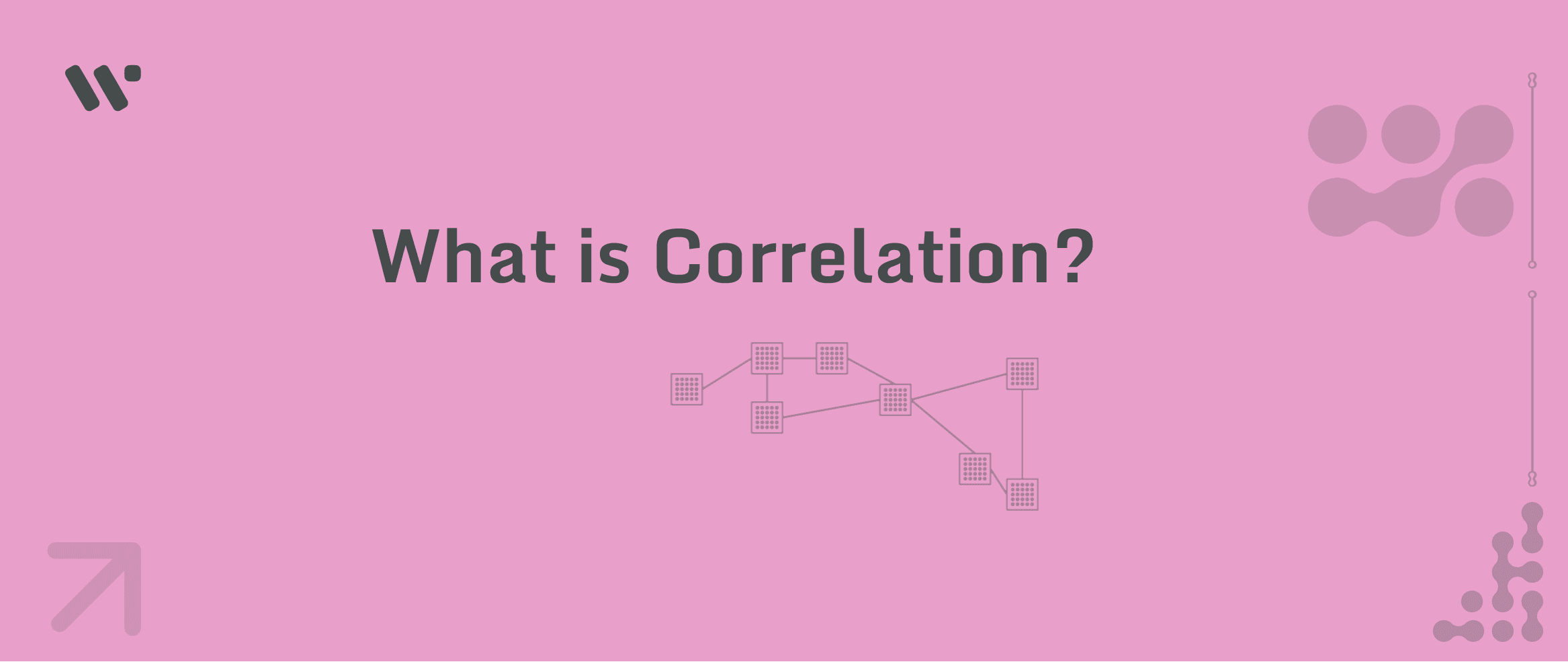