Matthew Webb
Mastering Web3.js and Web3.py for Blockchain Development
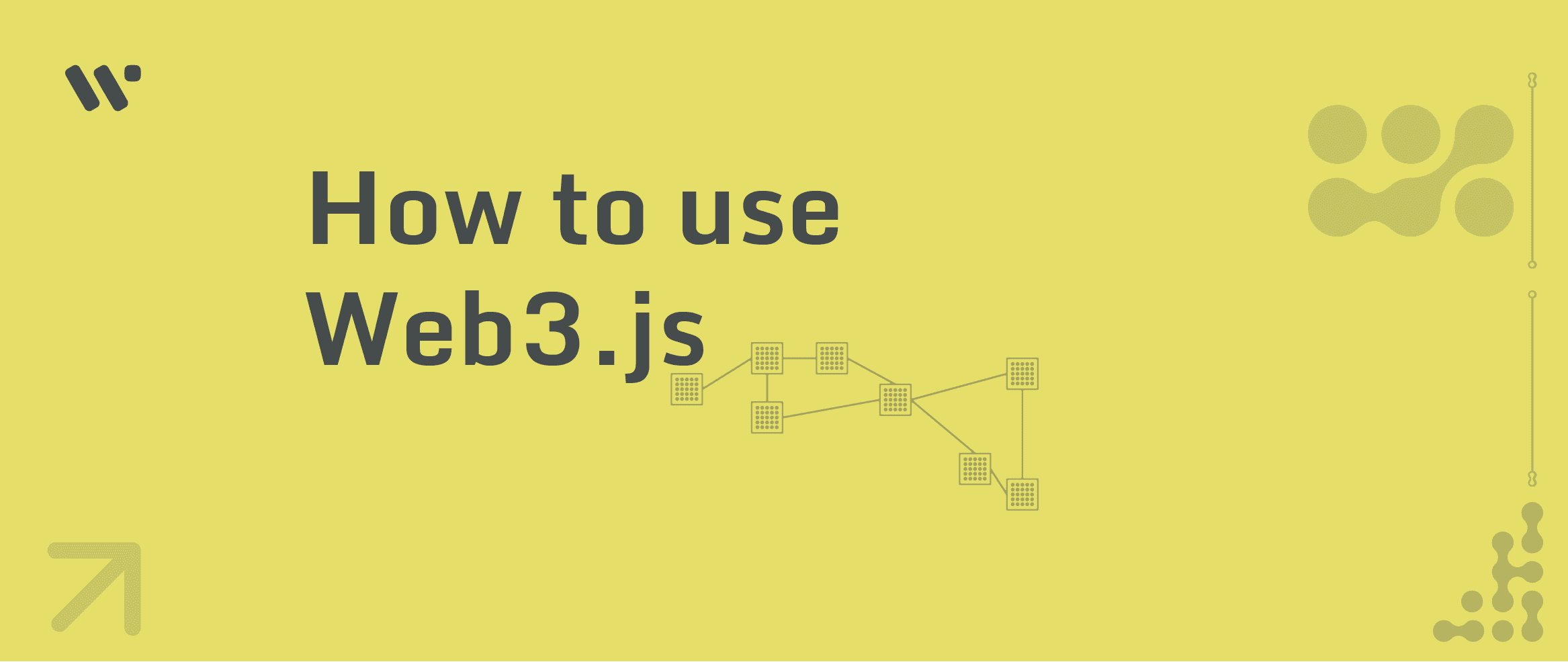
Disclaimer
This article is for educational purposes only and should not be considered as financial or investment advice. Always do your own research and consult with a qualified professional before making any investment decisions or engaging in blockchain development.
Introduction
In the world of blockchain technology, developers need robust tools to interact with decentralized networks. Two of the most popular libraries for this purpose are Web3.js for JavaScript developers and Web3.py for Python enthusiasts. These libraries serve as bridges between your applications and the blockchain, enabling you to create powerful decentralized applications (dApps) and interact with smart contracts seamlessly.
Blockchain technology, at its core, is about decentralization and trustless interactions. When we talk about "interacting with the blockchain," we're referring to actions like sending transactions, querying the state of the network, or executing functions on smart contracts. Web3 libraries abstract away much of the complexity involved in these interactions, providing developers with intuitive interfaces to build blockchain-powered applications.
In this comprehensive guide, we'll explore how to use Web3.js and Web3.py for blockchain development, focusing primarily on the Ethereum ecosystem. Whether you're a seasoned developer or just starting your journey in the blockchain space, this article will provide you with the knowledge and tools to build cutting-edge decentralized applications.
Getting Started with Web3.js
What is Web3.js?
Web3.js is a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket. It's the go-to library for JavaScript developers looking to build dApps on Ethereum or other compatible blockchains.
But what does this mean in practice? Essentially, Web3.js provides a way for your JavaScript code to communicate with the Ethereum blockchain. It allows you to do things like:
- Send Ether from one account to another
- Read and write data to smart contracts
- Deploy new smart contracts
- Sign messages and transactions
- Interact with the Ethereum Name Service (ENS)
All of these operations are abstracted into simple function calls, making it much easier for developers to build blockchain applications without needing to understand all the underlying cryptographic and networking details.
Installation and Setup
To get started with Web3.js, you'll need to have Node.js and npm (Node Package Manager) installed on your system. Once you have these prerequisites, you can install Web3.js using npm:
npm install web3
After installation, you can import Web3 into your JavaScript project:
const Web3 = require('web3');
This line imports the Web3 library, making all of its functions and utilities available in your project.
Connecting to an Ethereum Node
To interact with the Ethereum network, you need to connect to an Ethereum node. You can run your own node or use a service like Infura. Here's how to establish a connection:
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR-PROJECT-ID');
Replace 'YOUR-PROJECT-ID' with your actual Infura project ID or use the URL of your own Ethereum node.
What's happening here? You're creating a new Web3 instance and telling it how to connect to the Ethereum network. The URL you provide points to an Ethereum node (in this case, one hosted by Infura) that will relay your requests to the broader Ethereum network and return the results.
Basic Operations with Web3.js
Checking Account Balance
One of the most common operations is checking an account's balance. Here's how you can do it with Web3.js:
async function getBalance(address) {
const balance = await web3.eth.getBalance(address);
console.log(`Balance: ${web3.utils.fromWei(balance, 'ether')} ETH`);
}
getBalance('0x742d35Cc6634C0532925a3b844Bc454e4438f44e');
Let's break this down:
web3.eth.getBalance(address)
queries the Ethereum network for the balance of the specified address.- The balance is returned in wei, the smallest unit of Ether.
web3.utils.fromWei(balance, 'ether')
converts the balance from wei to Ether, which is more readable for humans.
This function is asynchronous because querying the blockchain takes time - we need to wait for the network to respond.
Sending Transactions
To send a transaction, you'll need to sign it with a private key. Here's a basic example:
async function sendTransaction(fromAddress, toAddress, amount, privateKey) {
const nonce = await web3.eth.getTransactionCount(fromAddress);
const gasPrice = await web3.eth.getGasPrice();
const txObject = {
nonce: web3.utils.toHex(nonce),
to: toAddress,
value: web3.utils.toHex(web3.utils.toWei(amount, 'ether')),
gasLimit: web3.utils.toHex(21000),
gasPrice: web3.utils.toHex(gasPrice)
};
const signedTx = await web3.eth.accounts.signTransaction(txObject, privateKey);
const receipt = await web3.eth.sendSignedTransaction(signedTx.rawTransaction);
console.log(`Transaction hash: ${receipt.transactionHash}`);
}
This function does several things:
- It gets the nonce (transaction count) for the sending address. This is required to prevent replay attacks.
- It fetches the current gas price from the network.
- It constructs a transaction object with all necessary fields.
- It signs the transaction with the provided private key.
- Finally, it sends the signed transaction to the network and waits for a receipt.
Remember, sending transactions requires Ether to pay for gas, and exposing private keys is a security risk. In a real application, you'd use more secure methods to manage private keys.
Interacting with Smart Contracts
Web3.js makes it easy to interact with smart contracts. First, you'll need the contract's ABI (Application Binary Interface) and address:
const contractABI = [...]; // Your contract ABI here
const contractAddress = '0x...'; // Your contract address here
const contract = new web3.eth.Contract(contractABI, contractAddress);
The ABI is a JSON representation of the contract's interface. It tells Web3.js what functions are available and how to call them. The address is where the contract is deployed on the Ethereum network.
Now you can call contract functions:
async function callContractFunction() {
const result = await contract.methods.someFunction().call();
console.log(`Function result: ${result}`);
}
This function calls a read-only (view) function on the contract. If you need to change the state of the contract (a write operation), you'd need to send a transaction instead of just calling the function.
Exploring Web3.py
What is Web3.py?
Web3.py is the Python equivalent of Web3.js. It provides a set of libraries to interact with Ethereum, making it the preferred choice for Python developers in the blockchain space.
Just like Web3.js, Web3.py allows you to connect to the Ethereum network, send transactions, interact with smart contracts, and more, all from within your Python applications.
Installation and Setup
To install Web3.py, you can use pip:
pip install web3
After installation, import Web3 in your Python script:
from web3 import Web3
This imports the main Web3 class, which you'll use to interact with the Ethereum network.
Connecting to an Ethereum Node
Similar to Web3.js, you need to connect to an Ethereum node:
w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR-PROJECT-ID'))
This creates a Web3 instance connected to the Ethereum mainnet via Infura. You can replace the URL with your own Ethereum node if you're running one.
Basic Operations with Web3.py
Checking Account Balance
Here's how to check an account's balance using Web3.py:
def get_balance(address):
balance = w3.eth.get_balance(address)
print(f"Balance: {w3.from_wei(balance, 'ether')} ETH")
get_balance('0x742d35Cc6634C0532925a3b844Bc454e4438f44e')
This function works similarly to its Web3.js counterpart:
w3.eth.get_balance(address)
fetches the balance from the Ethereum network.w3.from_wei(balance, 'ether')
converts the balance from wei to Ether.
Sending Transactions
Sending a transaction with Web3.py is similar to Web3.js:
from eth_account import Account
def send_transaction(from_address, to_address, amount, private_key):
nonce = w3.eth.get_transaction_count(from_address)
gas_price = w3.eth.gas_price
tx = {
'nonce': nonce,
'to': to_address,
'value': w3.to_wei(amount, 'ether'),
'gas': 21000,
'gasPrice': gas_price
}
signed_tx = Account.sign_transaction(tx, private_key)
tx_hash = w3.eth.send_raw_transaction(signed_tx.rawTransaction)
receipt = w3.eth.wait_for_transaction_receipt(tx_hash)
print(f"Transaction hash: {receipt.transactionHash.hex()}")
This function follows the same steps as the Web3.js version:
- Get the nonce for the sending address.
- Fetch the current gas price.
- Construct the transaction object.
- Sign the transaction with the private key.
- Send the transaction and wait for the receipt.
The main difference is in syntax and some function names, but the overall process is the same.
Interacting with Smart Contracts
To interact with smart contracts using Web3.py:
contract_abi = [...] # Your contract ABI here
contract_address = '0x...' # Your contract address here
contract = w3.eth.contract(address=contract_address, abi=contract_abi)
def call_contract_function():
result = contract.functions.someFunction().call()
print(f"Function result: {result}")
This setup is very similar to Web3.js. You create a contract instance using the ABI and address, then you can call functions on that contract. The .call()
method is used for read-only functions that don't change the contract's state.
Best Practices and Advanced Topics
Gas Optimization
When developing on Ethereum, gas optimization is crucial. Gas is the fuel that powers computations on the Ethereum network, and users have to pay for it. Therefore, optimizing gas usage can save your users money and make your dApps more efficient.
Always estimate gas costs before sending transactions:
// Web3.js
const gasEstimate = await contract.methods.someFunction().estimateGas();
# Web3.py
gas_estimate = contract.functions.someFunction().estimate_gas()
These methods simulate the transaction and return an estimate of how much gas it will consume. You can use this estimate to set an appropriate gas limit for your transaction.
Error Handling
Proper error handling is essential in blockchain development. Network issues, out-of-gas errors, and contract exceptions are all common in blockchain interactions. Always wrap your function calls in try-catch blocks:
// Web3.js
try {
const result = await contract.methods.someFunction().call();
} catch (error) {
console.error('An error occurred:', error);
}
# Web3.py
try:
result = contract.functions.someFunction().call()
except Exception as e:
print(f"An error occurred: {e}")
This allows you to gracefully handle errors and provide meaningful feedback to your users.
Event Listening
Both Web3.js and Web3.py allow you to listen for events emitted by smart contracts. This is crucial for building responsive dApps that can react to on-chain events in real-time.
// Web3.js
contract.events.SomeEvent({}, (error, event) => {
console.log('Event received:', event);
});
# Web3.py
event_filter = contract.events.SomeEvent.create_filter(fromBlock='latest')
for event in event_filter.get_new_entries():
print('Event received:', event)
In Web3.js, you can set up a callback function that will be called whenever the specified event is emitted. In Web3.py, you create a filter and then periodically check for new events.
Conclusion
Web3.js and Web3.py are powerful libraries that open up a world of possibilities in blockchain development. Whether you're building decentralized finance (DeFi) applications, non-fungible token (NFT) platforms, or other blockchain-based solutions, these libraries provide the tools you need to interact with Ethereum and other compatible networks.
As you continue your journey in blockchain development, remember to stay updated with the latest developments in the Ethereum ecosystem and the ongoing improvements to these libraries. The blockchain space is rapidly evolving, and staying informed is key to building secure and efficient decentralized applications.
Some key points to remember:
- Always prioritize security. When dealing with cryptocurrencies and smart contracts, even small bugs can lead to significant financial losses.
- Gas optimization is crucial for creating user-friendly dApps on Ethereum.
- Proper error handling can greatly improve the user experience of your dApps.
- Event listening allows your applications to react in real-time to on-chain events.
- Both Web3.js and Web3.py offer similar functionality, so choose based on your preferred programming language and ecosystem.
By mastering Web3.js and Web3.py, you're well on your way to becoming a proficient blockchain developer. These libraries abstract away much of the complexity of blockchain interactions, allowing you to focus on building innovative decentralized applications.
Remember, the best way to learn is by doing. Start with small projects, experiment with different features of these libraries, and gradually build up to more complex applications. Don't be afraid to dive into the documentation and explore advanced features as you become more comfortable with the basics.
Keep experimenting, building, and contributing to the decentralized future! The blockchain space is still in its early stages, and there's enormous potential for developers to make significant impacts. Whether you're interested in finance, gaming, social media, or any other field, blockchain technology offers new ways to approach old problems and create entirely new possibilities.
Happy coding, and welcome to the exciting world of blockchain development!
Related Posts
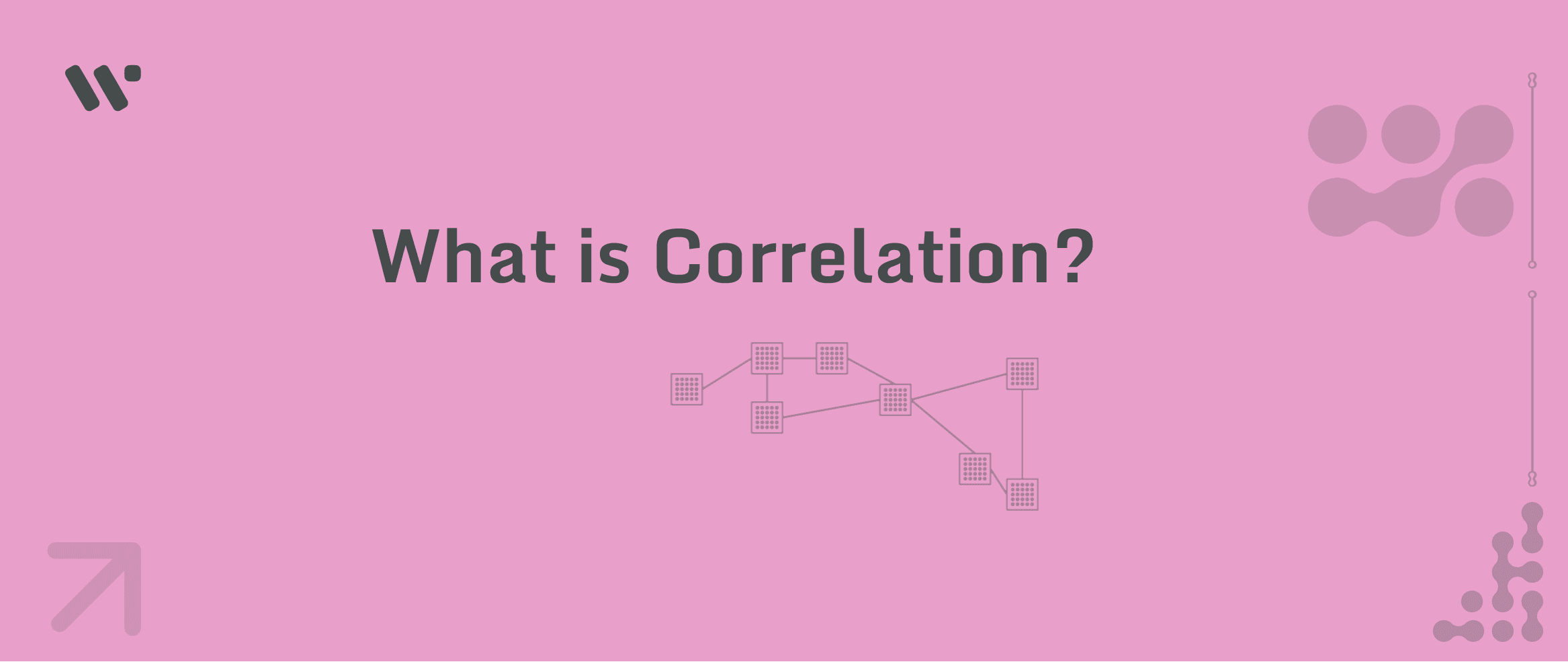